Quarkus & Couchbase: a simple REST example
CLOUD & DEVOPS
Quarkus & Couchbase: a simple REST example
event 20/04/2023
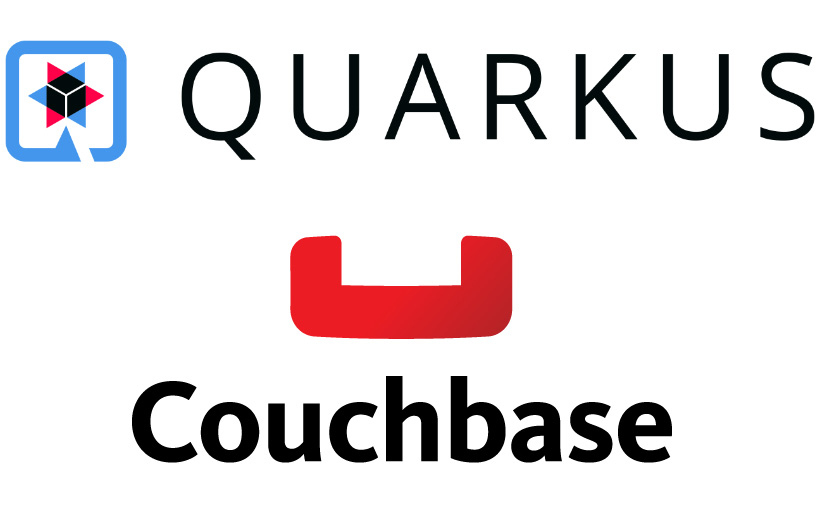
In one of the events I attended in the past months, I had the opportunity to meet some colleagues who work for Couchbase. I was familiar with the renowned product but had never had a chance to use it, so I asked them to briefly explain its features, and I must say it was interesting. I invited the guys to come to Extra Red and share their products with us, and they will indeed be guests at one of our Symposiums, a monthly event where we talk about technologies, methodologies, projects, and much more with all our colleagues.
For those who are not familiar, Couchbase is an open-source, distributed NoSQL database designed to provide fast, scalable, and flexible data management with high performance. It utilizes a document-based data model, where data is organized in JSON documents that can easily scale horizontally across multiple nodes. It is also capable of handling large amounts of data reliably and offers data redundancy and fault tolerance, thanks to its native integration with Kubernetes and Openshift. In fact, there is a dedicated operator that makes cluster creation extremely simple and fast.
I am particularly interested in the application aspect, especially the use of Couchbase as a database. That's why I started doing some usage tests with Quarkus, a Java cloud-native technology that we extensively use. The idea was to create a simple REST interface to write and read JSON files to the database. However, since I found very few examples, I decided to write this short article to share my experience, hoping it could be useful to others as well.
You can find the link to the repository on GitHub with the example code at the end.
As I mentioned, it is a simple project created using Quarkus' own Maven project generator. Then, I used the (still in alpha) "quarkus-couchbase" connection library by simply adding the following dependency to the pom file:
<dependency> <groupId>com.couchbase</groupId> <artifactId>quarkus-couchbase</artifactId> <version>1.0.0-alpha.1</version> </dependency>
As you can see in the library info, it is configured simply by adding the properties to the "application.properties" file:
quarkus.couchbase.connection-string=localhost quarkus.couchbase.username=username quarkus.couchbase.password=password
The usage of the library is quite simple, and you will find what I describe in the "QuarkusCouchbaseService" class, where the REST methods are defined:
The library allows us to inject the Cluster, from which we retrieve the bucket we want to read/write to.
- Through this, we retrieve the bucket on which we want to read/write.
- At this point, we use the 'get' and 'upsert' methods of the collection, respectively, to read and write the files we have received via REST
@Inject Cluster cluster; @ConfigProperty(name = "couchbase.bucketName") String couchbaseBucketName; @GET @Path("/getFile/{id}") @Produces(MediaType.APPLICATION_JSON) @Operation(summary = "Gets a file", description = "Retrieves a json file by id") @APIResponses(value = { @APIResponse(responseCode = "200", description = "Success", content = @Content(mediaType = "application/json")), @APIResponse(responseCode = "404", description="File not found", content = @Content(mediaType = "application/json")) }) public Response getFile(@PathParam("id") String id) { var bucket = cluster.bucket(couchbaseBucketName); var collection = bucket.defaultCollection(); try { return Response.ok(collection.get(id).contentAsObject().toString()).build(); } catch (DocumentNotFoundException e){ return Response.status(Response.Status.NOT_FOUND.getStatusCode(),"{\"error\":\"Not Found\"}").build(); } } @POST @Path("/sendFile") @Consumes(MediaType.APPLICATION_JSON) @Produces(MediaType.APPLICATION_JSON) @Operation(summary = "Store File", description = "Store a json file into database, return the id of the new element") @APIResponses(value = { @APIResponse(responseCode = "200", description = "Success", content = @Content(mediaType = "application/json")), @APIResponse(responseCode = "404", description="File not found", content = @Content(mediaType = "application/json")) }) public Response storeFile(String body) { var bucket = cluster.bucket(couchbaseBucketName); var collection = bucket.defaultCollection(); String id = UUID.randomUUID().toString(); JsonObject jsonObj = JsonObject.fromJson(body); MutationResult upsertResult = collection.upsert(id,jsonObj); return Response.ok("{\"id\":\"" + id +"\"}").build(); }
Note that for the insert case, I created a generic id, which should then be used as a parameter in the GET request to retrieve the specific JSON file. This is because the server does not generate an identifier automatically but needs to be explicitly specified during creation.
That's it! To perform various tests, I simply used the Couchbase Docker image available on Docker Hub. I ran the database locally using Docker, created a bucket, and configured the accesses as specified above.
At this point, you can write and retrieve files from the database, perhaps using Postman or simply with curl:
Write a file to the database:
curl --location --request POST 'http://localhost:8080/quarkus/sample/sendFile' \ --header 'Content-Type: application/json' \ --data-raw '{ "prova":123, "test":"stringa" }'
The response will be similar to this:
{ "id": "f8b980eb-1fa0-4070-bcd6-ff3fdce23faf" }
Now let's test retrieving the file:
curl --location --request GET 'http://localhost:8080/quarkus/sample/getFile/f8b980eb-1fa0-4070-bcd6-ff3fdce23faf' \ --data-raw ''
This will give us the following response:
{ "test": "stringa", "prova": 123 }
That's it! I hope this was helpful. If you have any suggestions or proposals, they are welcome, perhaps even an in-depth look at Quarkus, for example.
Useful Links:
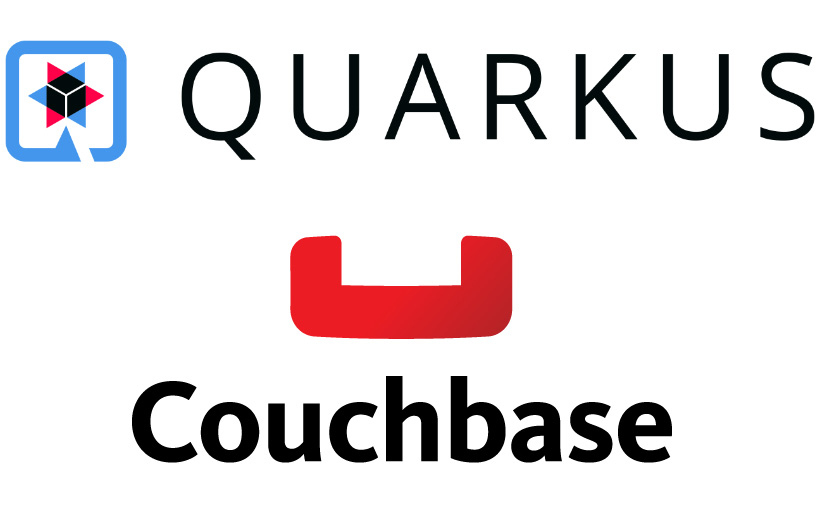
Failed to "?eval" string with this error: ---begin-message--- Syntax error in ?eval-ed string in line 1, column 718: Lexical error: encountered "u" (117), after "\"Extra\\". ---end-message--- The failing expression: ==> entitiesListJson?eval [in template "20097#20123#43230" at line 59, column 29] ---- FTL stack trace ("~" means nesting-related): - Failed at: #assign entities = entitiesListJson?eval [in template "20097#20123#43230" at line 59, column 9] ----
1<#-- Articoli in evidenza ADT -->
2<#assign portletBackground = "bg-red-white" />
3<#list portletPreferences?keys as key>
4 <#if key=="portletBackground">
5 <#assign portletBackground=portletPreferences[key]?first />
6 </#if>
7</#list>
8
9<#assign assetCategoryPropertyLocalService = serviceLocator.findService("com.liferay.asset.category.property.service.AssetCategoryPropertyLocalService") />
10<#assign categoryService = serviceLocator.findService("it.extrared.redweb.commons.service.CategoryService") />
11<#assign assetEntryLocalService=serviceLocator.findService("com.liferay.asset.kernel.service.AssetEntryLocalService") />
12<#assign dlFileEntryService = serviceLocator.findService('com.liferay.document.library.kernel.service.DLFileEntryService') />
13<#assign otherArticles = languageUtil.get(locale, "other-articles") />
14<#assign readMore = languageUtil.get(locale, "read-all") />
15
16<#assign redSimilarJournalArticle = serviceLocator.findService("it.extrared.redweb.commons.service.SimilarJournalArticle") />
17<#assign journalArticleLocalService = serviceLocator.findService("com.liferay.journal.service.JournalArticleLocalService") />
18<#assign dlAppServiceUtil = serviceLocator.findService("com.liferay.document.library.kernel.service.DLAppService")/>
19
20<#assign article = "" />
21<#assign urlTitle = "" />
22
23<#assign dirtyUrlTitle = themeDisplay.getURLCurrent() />
24<#assign curPage = 1 />
25
26<#if dirtyUrlTitle?contains("redirect")>
27 <#assign namespace = "_" + paramUtil.getString(request, "p_p_id") />
28 <#assign currentUrl = "_" + paramUtil.getString(request, namespace + "_redirect") />
29 <#assign urlTitle = currentUrl?split("/")?last />
30 <#assign curPage = paramUtil.getString(request, namespace + "_cur")/>
31<#elseif dirtyUrlTitle?contains("?wkrh___tabs1=properties")>
32 <#assign last = themeDisplay.getURLCurrent()?split("/")?last />
33 <#assign urlTitle = last?substring(0, last?index_of('?wkrh___tabs1=properties')) />
34<#elseif dirtyUrlTitle?contains("?")>
35 <#assign last = themeDisplay.getURLCurrent()?split("/")?last />
36 <#assign urlTitle = last?substring(0, last?index_of('?')) />
37<#else>
38 <#assign urlTitle = themeDisplay.getURLCurrent()?split("/")?last />
39</#if>
40
41
42<#assign logfactory = staticUtil["com.liferay.portal.kernel.log.LogFactoryUtil"] />
43<#assign log = logfactory.getLog("FreemarkerLog") />
44${log.info("urlTitle: " + urlTitle)}
45
46<#attempt>
47 <#assign article = journalArticleLocalService.getArticleByUrlTitle(groupId, urlTitle) />
48<#recover>
49</#attempt>
50
51<#assign entitiesListJson = "" />
52
53<#if article?? && article?has_content>
54 <#assign entitiesListJson = redSimilarJournalArticle.getSimilarJournalArticlesWithConfig(portletPreferences, article.articleId, groupId, false, curPage?number) />
55</#if>
56
57
58<#if entitiesListJson?? && entitiesListJson?has_content>
59 <#assign entities = entitiesListJson?eval />
60</#if>
61
62<#assign noResult = languageUtil.get(locale, "no-result") />
63
64<#if entities?has_content && entities?size gt 0>
65 <section class="container-fluid bg5percent">
66 <div class="swiper container ">
67 <p class="mb-5 text-muted text-uppercase">${otherArticles}</p>
68 <div class="swiper-wrapper align-items-center pt-lg-5 mb-lg-5">
69 <#list entities?keys as k>
70 <#assign entitiesGroup=entities[k] />
71 <#if entitiesGroup?size gt 0>
72 <#list entitiesGroup as array>
73 <div class="swiper-slide">
74 <#assign urlImage = "" />
75 <#assign altImage = "" />
76 <#if array["image"]?? && array["image"]?has_content>
77 <#assign docVal=array["image"]?eval />
78 <#if docVal.url?? && docVal.url?has_content>
79 <#assign urlImage = docVal.url />
80 </#if>
81 <#assign fileEntryId = docVal.fileEntryId />
82 </#if>
83
84 <#if docVal.title?? && docVal.title?has_content>
85 <#assign altImage = docVal.title />
86 </#if>
87
88 <#assign titolo = array["articleTitle"] />
89 <#assign categories = array["categoriesString"] />
90 <#assign displayPage = array["displayPage"] />
91 <#assign assetEntryId = array["assetEntryId"] />
92 <#assign assetEntry = assetEntryLocalService.getEntry(assetEntryId) />
93 <#assign categoriesList = assetEntry.getCategories() />
94
95 <div class="col-lg-9 col-9 col-sm-9 p-0">
96 <@adaptive_media_image["img"] fileVersion=dlAppServiceUtil.getFileEntry(fileEntryId?number).getFileVersion() class="mb-4" width="100%" height="auto" alt="${altImage}"/>
97 <#--
98 <img width="100%" class="mb-4" height="auto" src="${urlImage}" alt="${altImage}" title="${titolo}">
99 -->
100 </div>
101 <div class="card-body pt-2">
102 <#if categoriesList?? && categoriesList?has_content>
103 <div class="card-subtitle text-muted">
104 <#assign categoriesString = "" />
105 <#list categoriesList as c>
106 <#assign hasProperty = categoryService.hasCategoryProperty(c.getCategoryId(), "type") />
107 <#if !hasProperty>
108 <#if !categoriesString?has_content>
109 <#assign categoriesString = c.getTitle(locale)?upper_case />
110 <#else>
111 <#assign categoriesString = categoriesString + ", " + c.getTitle(locale)?upper_case />
112 </#if>
113 </#if>
114 <#--
115 <#attempt>
116 <#assign typeProperty = assetCategoryPropertyLocalService.fetchCategoryProperty(c.categoryId?long, "type") />
117 <#recover>
118 <#if !categoriesString?has_content>
119 <#assign categoriesString = c.getTitle(locale)?upper_case />
120 <#else>
121 <#assign categoriesString = categoriesString + ", " + c.getTitle(locale)?upper_case />
122 </#if>
123 </#attempt>
124 -->
125 </#list>
126
127 ${categoriesString}
128 </div>
129 </#if>
130 <a href="${displayPage}" target="_self" title="${titolo}">
131 <h3 class="m-0">${titolo}</h3>
132 </a>
133 </div>
134 </div>
135 </#list>
136 <#else>
137 <div class="container">
138 <h2 class="m-0">${noResult}</h2>
139 </div>
140 </#if>
141 </#list>
142 </div>
143 <a data-aos="fade-right" class="pt-5 align-middle" style="display: block" href="/blog">
144 <i class="align-middle me-2 material-icons-outlined">explore</i>
145 <span class="align-middle">${readMore?capitalize}</span>
146 </a>
147 </div>
148 </section>
149</#if>